Lean and Agile Development has been around for many years now and numerous practices have evolved around various phases of software development. There has been a proliferation of consulting services around agile and lean. Fortunately or unfortunately, not many methodologies have evolved - apart from XP and Scrum.
Most articles or books on Agile development start with a reference to the Agile Manifesto as a precursor to the practices followed. But to obtain a better understanding why agile practices are followed, we need to understand why individuals and interactions are valued over processes and tools, why working software is preferred over comprehensive documentation, why responding to change is more important than following a plan and why customer collaboration is considered paramount.
It is common sense that businesses exist to maximize profit, but what is uncommon about some of the software methods of the past (Six Sigma, ISO 9000) is how following those would result in better business profitability. ISO and Six-Sigma touted that improving quality and reducing defects would result in better customer satisfaction and higher profit. But a defect-free product that arrived years late does not improve profit.
Lean thinking and TPS is adopted to make sure products are delivered with reduced cycle times, but the base metrics are derived from the time-cost-quality triangle and optimizing one usually results in sub-par performance in another. To run an optimum business, we need a metric that follows the principle of optimal-substructure, that can be applied to all sub-components of the business and optimizing this metric in each area should help us optimize the metric for the whole business.
It is a fundamental fact that ‘asymmetric information’ leads to profitability. Insider trading in stocks, counting in card games, testing in drug research, A/B testing of web products, price comparison in online shopping, and many more such instances try to improve our ability to have better information and ultimately profit from it. Better information results in better decision making and higher profit. Information that is available to us much before it is available to the rest of the world can be considered asymmetric and enhances competitive advantage. In the software development process, the ‘product’ or outcome of various activities is in fact information in various forms - requirement documents, design documents, code, test specifications, reports, and so on. In the context of a business unit developing software one can think of better information delivered faster to the customer as an optimal metric that can be used to measure each individual activity We can call this metric Information Rate.
When we think of the software development process as an entire system, the best form of information one can deliver to the customer is actual working software. During the process of developing working software (information), there may be opportunities to improve the quality of this information by continuously interacting (customer collaboration) with the customer. If the customer thinks there is better opportunity for generating better information by introducing new inputs, one must respond to changes. We can now understand how meaningless it would be to deliver information that is outdated (working software that requires change). Lastly delivering software requires a set of steps where information is handed over by individuals from one step to next. For each such step, the individuals from the previous step act as customers and hence to improve customer collaboration, one needs to improve on individuals and interactions.
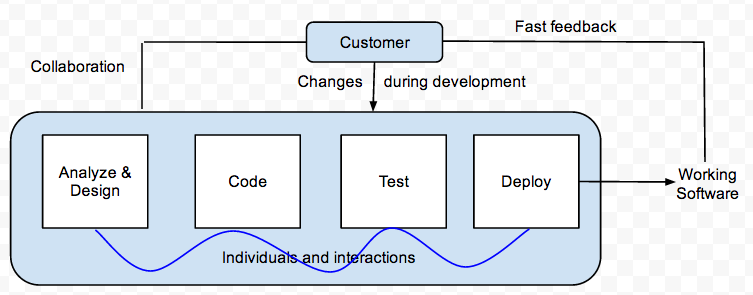
One can thus see how the principles of the agile manifesto directly lead to the generation of better, faster asymmetric information and has a direct impact on business profitability. This informational view of software is a paradigm shift in the way we approach software quality. It is no more necessary for working software in production to be perfect from day one, what is more important is for the software to be usable and functional, and most importantly to deliver the best information of how it needs to change to meet future requirements !!